This is the multi-page printable view of this section. Click here to print.
Integration tutorials
- 1: PyTorch
- 2: PyTorch Lightning
- 3: Hugging Face
- 4: TensorFlow
- 5: TensorFlow Sweeps
- 6: 3D brain tumor segmentation with MONAI
- 7: Keras
- 8: Keras models
- 9: Keras tables
- 10: XGBoost Sweeps
1 - PyTorch
Use Weights & Biases for machine learning experiment tracking, dataset versioning, and project collaboration.

What this notebook covers
We show you how to integrate Weights & Biases with your PyTorch code to add experiment tracking to your pipeline.
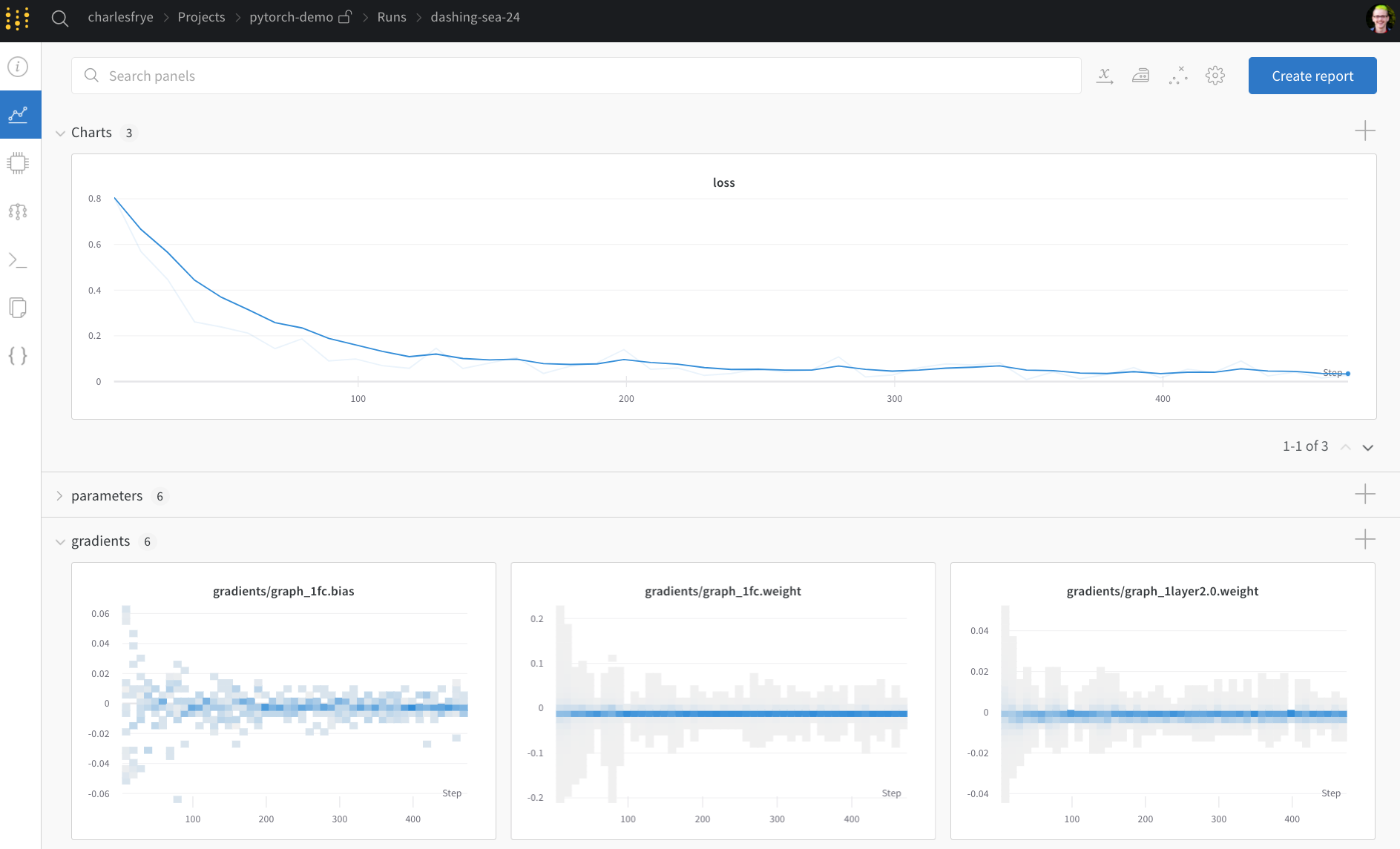
Follow along with a video tutorial.
Note: Sections starting with Step are all you need to integrate W&B in an existing pipeline. The rest just loads data and defines a model.
Install, import, and log in
Step 0: Install W&B
To get started, we’ll need to get the library.
wandb
is easily installed using pip
.
Step 1: Import W&B and Login
In order to log data to our web service, you’ll need to log in.
If this is your first time using W&B, you’ll need to sign up for a free account at the link that appears.
import wandb
wandb.login()
Define the Experiment and Pipeline
Track metadata and hyperparameters with wandb.init
Programmatically, the first thing we do is define our experiment: what are the hyperparameters? what metadata is associated with this run?
It’s a pretty common workflow to store this information in a config
dictionary
(or similar object)
and then access it as needed.
For this example, we’re only letting a few hyperparameters vary
and hand-coding the rest.
But any part of your model can be part of the config
.
We also include some metadata: we’re using the MNIST dataset and a convolutional architecture. If we later work with, say, fully connected architectures on CIFAR in the same project, this will help us separate our runs.
Now, let’s define the overall pipeline, which is pretty typical for model-training:
- we first
make
a model, plus associated data and optimizer, then - we
train
the model accordingly and finally test
it to see how training went.
We’ll implement these functions below.
The only difference here from a standard pipeline
is that it all occurs inside the context of wandb.init
.
Calling this function sets up a line of communication
between your code and our servers.
Passing the config
dictionary to wandb.init
immediately logs all that information to us,
so you’ll always know what hyperparameter values
you set your experiment to use.
To ensure the values you chose and logged are always the ones that get used
in your model, we recommend using the wandb.config
copy of your object.
Check the definition of make
below to see some examples.
Side Note: We take care to run our code in separate processes, so that any issues on our end (such as if a giant sea monster attacks our data centers) don’t crash your code. Once the issue is resolved, such as when the Kraken returns to the deep, you can log the data with
wandb sync
.
Define the Data Loading and Model
Now, we need to specify how the data is loaded and what the model looks like.
This part is very important, but it’s
no different from what it would be without wandb
,
so we won’t dwell on it.
Defining the model is normally the fun part.
But nothing changes with wandb
,
so we’re gonna stick with a standard ConvNet architecture.
Don’t be afraid to mess around with this and try some experiments – all your results will be logged on wandb.ai.
Define Training Logic
Moving on in our model_pipeline
, it’s time to specify how we train
.
Two wandb
functions come into play here: watch
and log
.
Track gradients with wandb.watch
and everything else with wandb.log
wandb.watch
will log the gradients and the parameters of your model,
every log_freq
steps of training.
All you need to do is call it before you start training.
The rest of the training code remains the same:
we iterate over epochs and batches,
running forward and backward passes
and applying our optimizer
.
The only difference is in the logging code:
where previously you might have reported metrics by printing to the terminal,
now you pass the same information to wandb.log
.
wandb.log
expects a dictionary with strings as keys.
These strings identify the objects being logged, which make up the values.
You can also optionally log which step
of training you’re on.
Side Note: I like to use the number of examples the model has seen, since this makes for easier comparison across batch sizes, but you can use raw steps or batch count. For longer training runs, it can also make sense to log by
epoch
.
Define Testing Logic
Once the model is done training, we want to test it: run it against some fresh data from production, perhaps, or apply it to some hand-curated examples.
(Optional) Call wandb.save
This is also a great time to save the model’s architecture
and final parameters to disk.
For maximum compatibility, we’ll export
our model in the
Open Neural Network eXchange (ONNX) format.
Passing that filename to wandb.save
ensures that the model parameters
are saved to W&B’s servers: no more losing track of which .h5
or .pb
corresponds to which training runs.
For more advanced wandb
features for storing, versioning, and distributing
models, check out our Artifacts tools.
Run training and watch your metrics live on wandb.ai
Now that we’ve defined the whole pipeline and slipped in those few lines of W&B code, we’re ready to run our fully tracked experiment.
We’ll report a few links to you: our documentation, the Project page, which organizes all the runs in a project, and the Run page, where this run’s results will be stored.
Navigate to the Run page and check out these tabs:
- Charts, where the model gradients, parameter values, and loss are logged throughout training
- System, which contains a variety of system metrics, including Disk I/O utilization, CPU and GPU metrics (watch that temperature soar), and more
- Logs, which has a copy of anything pushed to standard out during training
- Files, where, once training is complete, you can click on the
model.onnx
to view our network with the Netron model viewer.
Once the run in finished, when the with wandb.init
block exits,
we’ll also print a summary of the results in the cell output.
Test Hyperparameters with Sweeps
We only looked at a single set of hyperparameters in this example. But an important part of most ML workflows is iterating over a number of hyperparameters.
You can use Weights & Biases Sweeps to automate hyperparameter testing and explore the space of possible models and optimization strategies.
Check out Hyperparameter Optimization in PyTorch using W&B Sweeps
Running a hyperparameter sweep with Weights & Biases is very easy. There are just 3 simple steps:
-
Define the sweep: We do this by creating a dictionary or a YAML file that specifies the parameters to search through, the search strategy, the optimization metric et all.
-
Initialize the sweep:
sweep_id = wandb.sweep(sweep_config)
-
Run the sweep agent:
wandb.agent(sweep_id, function=train)
That’s all there is to running a hyperparameter sweep.
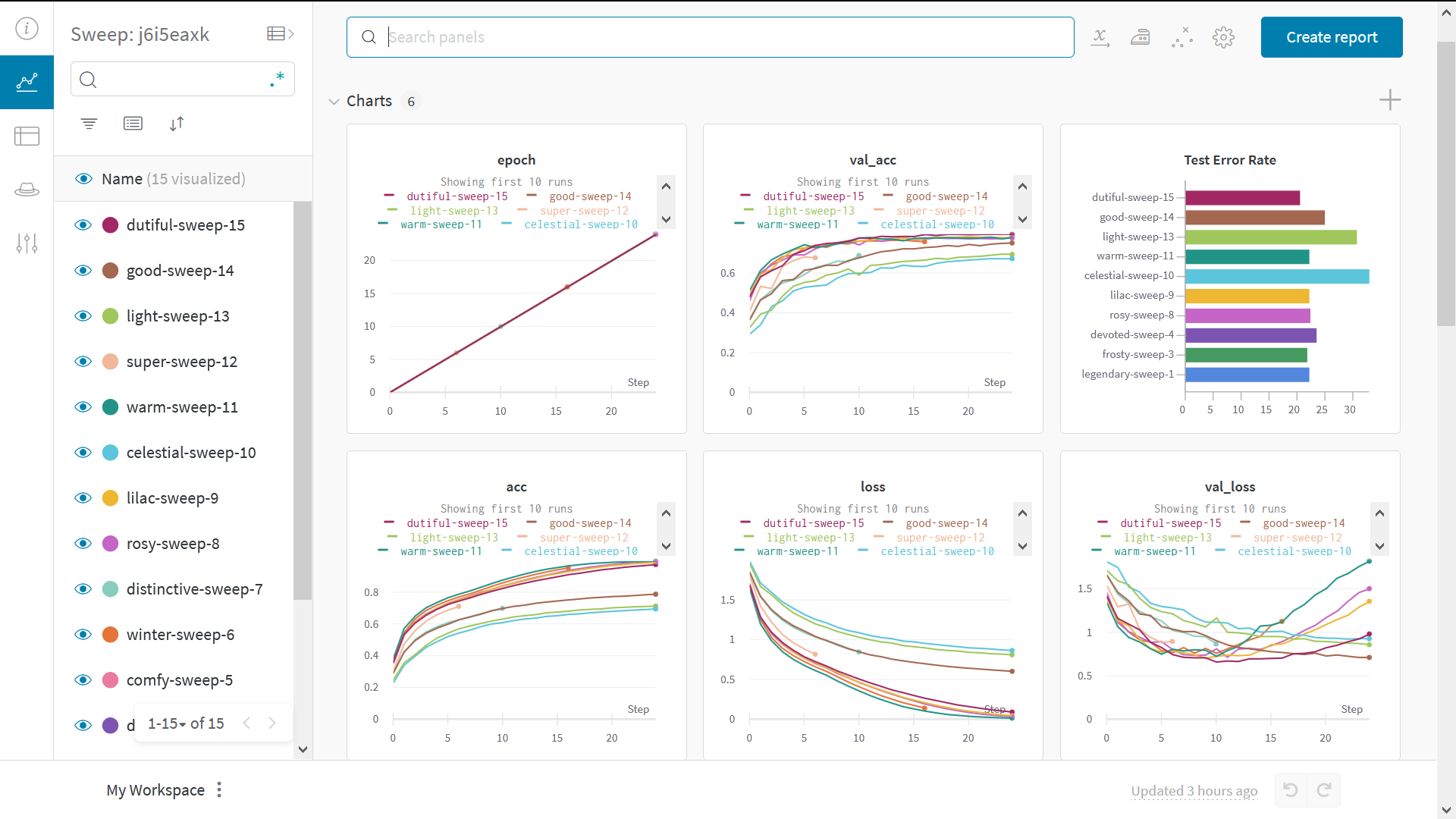
Example Gallery
See examples of projects tracked and visualized with W&B in our Gallery →
Advanced Setup
- Environment variables: Set API keys in environment variables so you can run training on a managed cluster.
- Offline mode: Use
dryrun
mode to train offline and sync results later. - On-prem: Install W&B in a private cloud or air-gapped servers in your own infrastructure. We have local installations for everyone from academics to enterprise teams.
- Sweeps: Set up hyperparameter search quickly with our lightweight tool for tuning.
2 - PyTorch Lightning
We will build an image classification pipeline using PyTorch Lightning. We will follow this style guide to increase the readability and reproducibility of our code. A cool explanation of this available here.Setting up PyTorch Lightning and W&B
For this tutorial, we need PyTorch Lightning and Weights and Biases.
Now you’ll need to log in to your wandb account.
wandb.login()
DataModule - The Data Pipeline we Deserve
DataModules are a way of decoupling data-related hooks from the LightningModule so you can develop dataset agnostic models.
It organizes the data pipeline into one shareable and reusable class. A datamodule encapsulates the five steps involved in data processing in PyTorch:
- Download / tokenize / process.
- Clean and (maybe) save to disk.
- Load inside Dataset.
- Apply transforms (rotate, tokenize, etc…).
- Wrap inside a DataLoader.
Learn more about datamodules here. Let’s build a datamodule for the Cifar-10 dataset.
class CIFAR10DataModule(pl.LightningDataModule):
def __init__(self, batch_size, data_dir: str = './'):
super().__init__()
self.data_dir = data_dir
self.batch_size = batch_size
self.transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])
self.num_classes = 10
def prepare_data(self):
CIFAR10(self.data_dir, train=True, download=True)
CIFAR10(self.data_dir, train=False, download=True)
def setup(self, stage=None):
# Assign train/val datasets for use in dataloaders
if stage == 'fit' or stage is None:
cifar_full = CIFAR10(self.data_dir, train=True, transform=self.transform)
self.cifar_train, self.cifar_val = random_split(cifar_full, [45000, 5000])
# Assign test dataset for use in dataloader(s)
if stage == 'test' or stage is None:
self.cifar_test = CIFAR10(self.data_dir, train=False, transform=self.transform)
def train_dataloader(self):
return DataLoader(self.cifar_train, batch_size=self.batch_size, shuffle=True)
def val_dataloader(self):
return DataLoader(self.cifar_val, batch_size=self.batch_size)
def test_dataloader(self):
return DataLoader(self.cifar_test, batch_size=self.batch_size)
Callbacks
A callback is a self-contained program that can be reused across projects. PyTorch Lightning comes with few built-in callbacks which are regularly used. Learn more about callbacks in PyTorch Lightning here.
Built-in Callbacks
In this tutorial, we will use Early Stopping and Model Checkpoint built-in callbacks. They can be passed to the Trainer
.
Custom Callbacks
If you are familiar with Custom Keras callback, the ability to do the same in your PyTorch pipeline is just a cherry on the cake.
Since we are performing image classification, the ability to visualize the model’s predictions on some samples of images can be helpful. This in the form of a callback can help debug the model at an early stage.
class ImagePredictionLogger(pl.callbacks.Callback):
def __init__(self, val_samples, num_samples=32):
super().__init__()
self.num_samples = num_samples
self.val_imgs, self.val_labels = val_samples
def on_validation_epoch_end(self, trainer, pl_module):
# Bring the tensors to CPU
val_imgs = self.val_imgs.to(device=pl_module.device)
val_labels = self.val_labels.to(device=pl_module.device)
# Get model prediction
logits = pl_module(val_imgs)
preds = torch.argmax(logits, -1)
# Log the images as wandb Image
trainer.logger.experiment.log({
"examples":[wandb.Image(x, caption=f"Pred:{pred}, Label:{y}")
for x, pred, y in zip(val_imgs[:self.num_samples],
preds[:self.num_samples],
val_labels[:self.num_samples])]
})
LightningModule - Define the System
The LightningModule defines a system and not a model. Here a system groups all the research code into a single class to make it self-contained. LightningModule
organizes your PyTorch code into 5 sections:
- Computations (
__init__
). - Train loop (
training_step
) - Validation loop (
validation_step
) - Test loop (
test_step
) - Optimizers (
configure_optimizers
)
One can thus build a dataset agnostic model that can be easily shared. Let’s build a system for Cifar-10 classification.
class LitModel(pl.LightningModule):
def __init__(self, input_shape, num_classes, learning_rate=2e-4):
super().__init__()
# log hyperparameters
self.save_hyperparameters()
self.learning_rate = learning_rate
self.conv1 = nn.Conv2d(3, 32, 3, 1)
self.conv2 = nn.Conv2d(32, 32, 3, 1)
self.conv3 = nn.Conv2d(32, 64, 3, 1)
self.conv4 = nn.Conv2d(64, 64, 3, 1)
self.pool1 = torch.nn.MaxPool2d(2)
self.pool2 = torch.nn.MaxPool2d(2)
n_sizes = self._get_conv_output(input_shape)
self.fc1 = nn.Linear(n_sizes, 512)
self.fc2 = nn.Linear(512, 128)
self.fc3 = nn.Linear(128, num_classes)
self.accuracy = Accuracy(task='multiclass', num_classes=num_classes)
# returns the size of the output tensor going into Linear layer from the conv block.
def _get_conv_output(self, shape):
batch_size = 1
input = torch.autograd.Variable(torch.rand(batch_size, *shape))
output_feat = self._forward_features(input)
n_size = output_feat.data.view(batch_size, -1).size(1)
return n_size
# returns the feature tensor from the conv block
def _forward_features(self, x):
x = F.relu(self.conv1(x))
x = self.pool1(F.relu(self.conv2(x)))
x = F.relu(self.conv3(x))
x = self.pool2(F.relu(self.conv4(x)))
return x
# will be used during inference
def forward(self, x):
x = self._forward_features(x)
x = x.view(x.size(0), -1)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = F.log_softmax(self.fc3(x), dim=1)
return x
def training_step(self, batch, batch_idx):
x, y = batch
logits = self(x)
loss = F.nll_loss(logits, y)
# training metrics
preds = torch.argmax(logits, dim=1)
acc = self.accuracy(preds, y)
self.log('train_loss', loss, on_step=True, on_epoch=True, logger=True)
self.log('train_acc', acc, on_step=True, on_epoch=True, logger=True)
return loss
def validation_step(self, batch, batch_idx):
x, y = batch
logits = self(x)
loss = F.nll_loss(logits, y)
# validation metrics
preds = torch.argmax(logits, dim=1)
acc = self.accuracy(preds, y)
self.log('val_loss', loss, prog_bar=True)
self.log('val_acc', acc, prog_bar=True)
return loss
def test_step(self, batch, batch_idx):
x, y = batch
logits = self(x)
loss = F.nll_loss(logits, y)
# validation metrics
preds = torch.argmax(logits, dim=1)
acc = self.accuracy(preds, y)
self.log('test_loss', loss, prog_bar=True)
self.log('test_acc', acc, prog_bar=True)
return loss
def configure_optimizers(self):
optimizer = torch.optim.Adam(self.parameters(), lr=self.learning_rate)
return optimizer
Train and Evaluate
Now that we have organized our data pipeline using DataModule
and model architecture+training loop using LightningModule
, the PyTorch Lightning Trainer
automates everything else for us.
The Trainer automates:
- Epoch and batch iteration
- Calling of
optimizer.step()
,backward
,zero_grad()
- Calling of
.eval()
, enabling/disabling grads - Saving and loading weights
- Weights and Biases logging
- Multi-GPU training support
- TPU support
- 16-bit training support
dm = CIFAR10DataModule(batch_size=32)
# To access the x_dataloader we need to call prepare_data and setup.
dm.prepare_data()
dm.setup()
# Samples required by the custom ImagePredictionLogger callback to log image predictions.
val_samples = next(iter(dm.val_dataloader()))
val_imgs, val_labels = val_samples[0], val_samples[1]
val_imgs.shape, val_labels.shape
model = LitModel((3, 32, 32), dm.num_classes)
# Initialize wandb logger
wandb_logger = WandbLogger(project='wandb-lightning', job_type='train')
# Initialize Callbacks
early_stop_callback = pl.callbacks.EarlyStopping(monitor="val_loss")
checkpoint_callback = pl.callbacks.ModelCheckpoint()
# Initialize a trainer
trainer = pl.Trainer(max_epochs=2,
logger=wandb_logger,
callbacks=[early_stop_callback,
ImagePredictionLogger(val_samples),
checkpoint_callback],
)
# Train the model
trainer.fit(model, dm)
# Evaluate the model on the held-out test set ⚡⚡
trainer.test(dataloaders=dm.test_dataloader())
# Close wandb run
wandb.finish()
Final Thoughts
I come from the TensorFlow/Keras ecosystem and find PyTorch a bit overwhelming even though it’s an elegant framework. Just my personal experience though. While exploring PyTorch Lightning, I realized that almost all of the reasons that kept me away from PyTorch is taken care of. Here’s a quick summary of my excitement:
- Then: Conventional PyTorch model definition used to be all over the place. With the model in some
model.py
script and the training loop in thetrain.py
file. It was a lot of looking back and forth to understand the pipeline. - Now: The
LightningModule
acts as a system where the model is defined along with thetraining_step
,validation_step
, etc. Now it’s modular and shareable. - Then: The best part about TensorFlow/Keras is the input data pipeline. Their dataset catalog is rich and growing. PyTorch’s data pipeline used to be the biggest pain point. In normal PyTorch code, the data download/cleaning/preparation is usually scattered across many files.
- Now: The DataModule organizes the data pipeline into one shareable and reusable class. It’s simply a collection of a
train_dataloader
,val_dataloader
(s),test_dataloader
(s) along with the matching transforms and data processing/downloads steps required. - Then: With Keras, one can call
model.fit
to train the model andmodel.predict
to run inference on.model.evaluate
offered a good old simple evaluation on the test data. This is not the case with PyTorch. One will usually find separatetrain.py
andtest.py
files. - Now: With the
LightningModule
in place, theTrainer
automates everything. One needs to just calltrainer.fit
andtrainer.test
to train and evaluate the model. - Then: TensorFlow loves TPU, PyTorch…
- Now: With PyTorch Lightning, it’s so easy to train the same model with multiple GPUs and even on TPU.
- Then: I am a big fan of Callbacks and prefer writing custom callbacks. Something as trivial as Early Stopping used to be a point of discussion with conventional PyTorch.
- Now: With PyTorch Lightning using Early Stopping and Model Checkpointing is a piece of cake. I can even write custom callbacks.
🎨 Conclusion and Resources
I hope you find this report helpful. I will encourage to play with the code and train an image classifier with a dataset of your choice.
Here are some resources to learn more about PyTorch Lightning:
- Step-by-step walk-through - This is one of the official tutorials. Their documentation is really well written and I highly encourage it as a good learning resource.
- Use Pytorch Lightning with Weights & Biases - This is a quick colab that you can run through to learn more about how to use W&B with PyTorch Lightning.
3 - Hugging Face
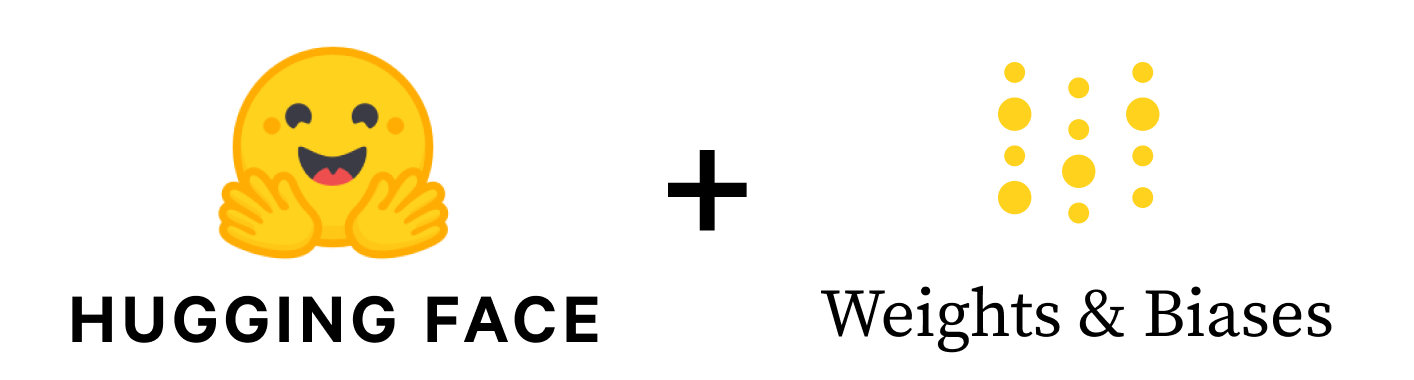
Compare hyperparameters, output metrics, and system stats like GPU utilization across your models.
Why should I use W&B?

- Unified dashboard: Central repository for all your model metrics and predictions
- Lightweight: No code changes required to integrate with Hugging Face
- Accessible: Free for individuals and academic teams
- Secure: All projects are private by default
- Trusted: Used by machine learning teams at OpenAI, Toyota, Lyft and more
Think of W&B like GitHub for machine learning models— save machine learning experiments to your private, hosted dashboard. Experiment quickly with the confidence that all the versions of your models are saved for you, no matter where you’re running your scripts.
W&B lightweight integrations works with any Python script, and all you need to do is sign up for a free W&B account to start tracking and visualizing your models.
In the Hugging Face Transformers repo, we’ve instrumented the Trainer to automatically log training and evaluation metrics to W&B at each logging step.
Here’s an in depth look at how the integration works: Hugging Face + W&B Report.
Install, import, and log in
Install the Hugging Face and Weights & Biases libraries, and the GLUE dataset and training script for this tutorial.
- Hugging Face Transformers: Natural language models and datasets
- Weights & Biases: Experiment tracking and visualization
- GLUE dataset: A language understanding benchmark dataset
- GLUE script: Model training script for sequence classification
Before continuing, sign up for a free account.
Put in your API key
Once you’ve signed up, run the next cell and click on the link to get your API key and authenticate this notebook.
Optionally, we can set environment variables to customize W&B logging. See documentation.
Train the model
Next, call the downloaded training script run_glue.py and see training automatically get tracked to the Weights & Biases dashboard. This script fine-tunes BERT on the Microsoft Research Paraphrase Corpus— pairs of sentences with human annotations indicating whether they are semantically equivalent.
Visualize results in dashboard
Click the link printed out above, or go to wandb.ai to see your results stream in live. The link to see your run in the browser will appear after all the dependencies are loaded. Look for the following output: “wandb: 🚀 View run at [URL to your unique run]”
Visualize Model Performance It’s easy to look across dozens of experiments, zoom in on interesting findings, and visualize highly dimensional data.
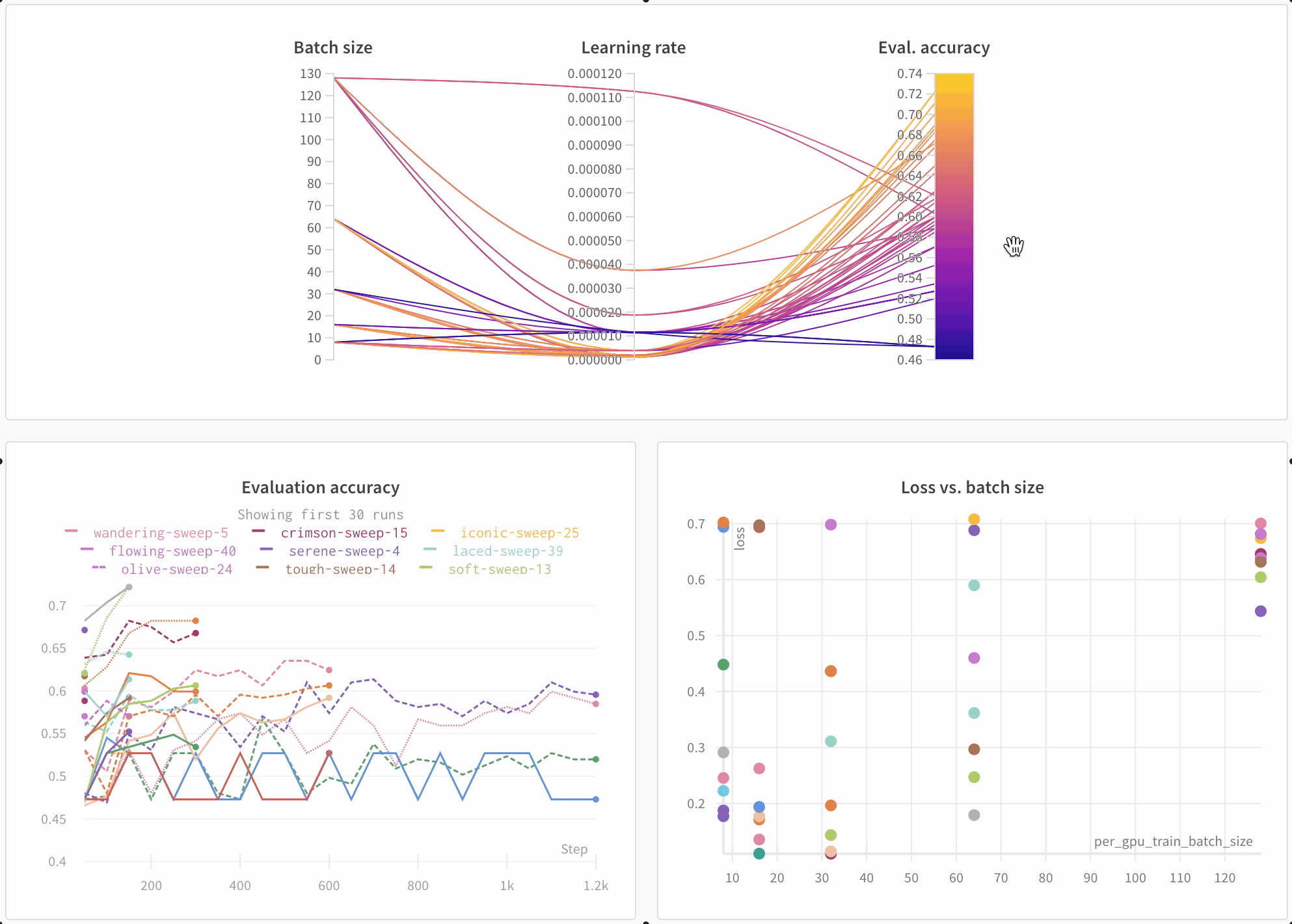
Compare Architectures Here’s an example comparing BERT vs DistilBERT. It’s easy to see how different architectures effect the evaluation accuracy throughout training with automatic line plot visualizations.
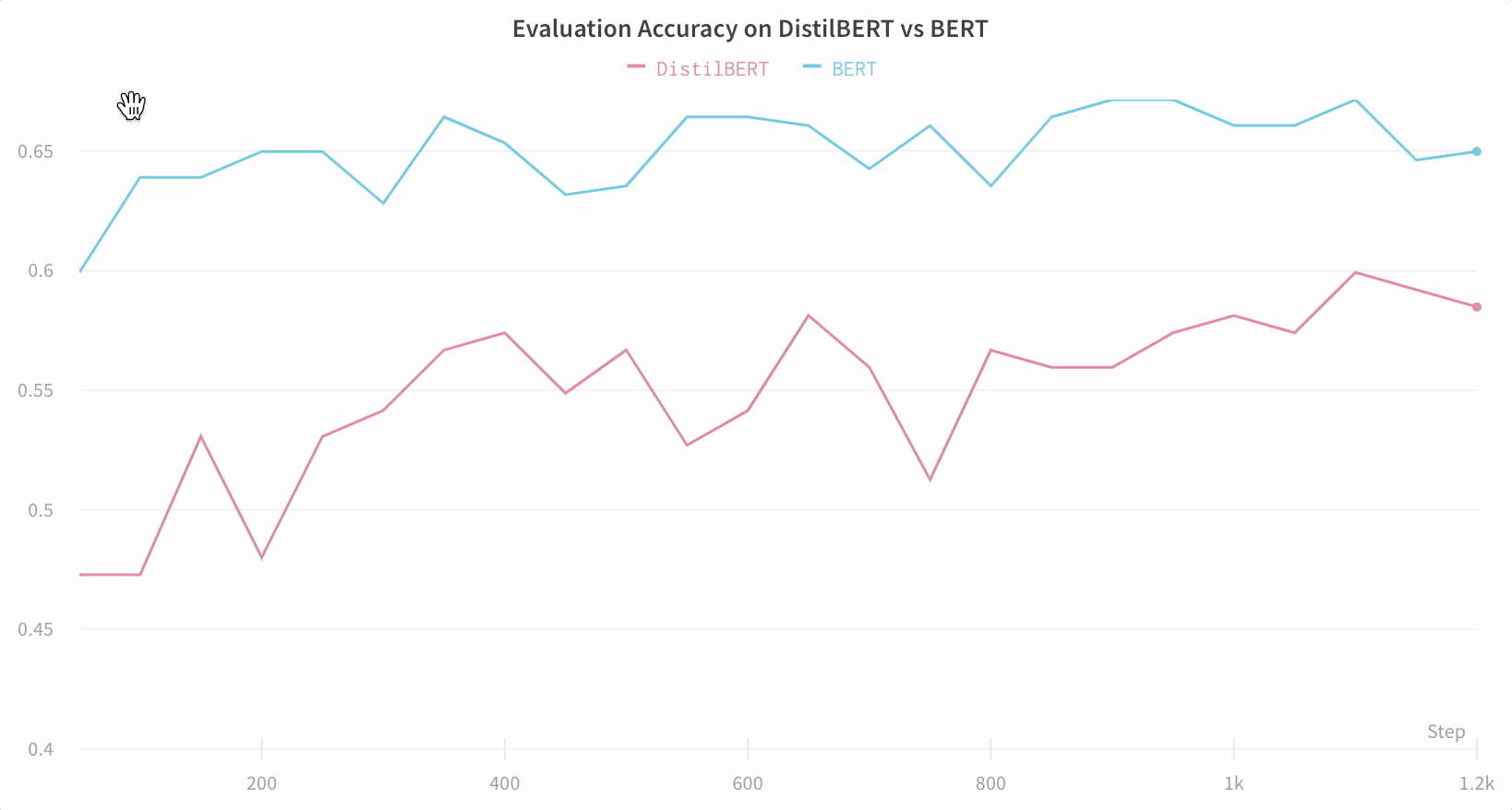
Track key information effortlessly by default
Weights & Biases saves a new run for each experiment. Here’s the information that gets saved by default:
- Hyperparameters: Settings for your model are saved in Config
- Model Metrics: Time series data of metrics streaming in are saved in Log
- Terminal Logs: Command line outputs are saved and available in a tab
- System Metrics: GPU and CPU utilization, memory, temperature etc.
Learn more
- Documentation: docs on the Weights & Biases and Hugging Face integration
- Videos: tutorials, interviews with practitioners, and more on our YouTube channel
- Contact: Message us at contact@wandb.com with questions
4 - TensorFlow
What this notebook covers
- Easy integration of Weights and Biases with your TensorFlow pipeline for experiment tracking.
- Computing metrics with
keras.metrics
- Using
wandb.log
to log those metrics in your custom training loop.
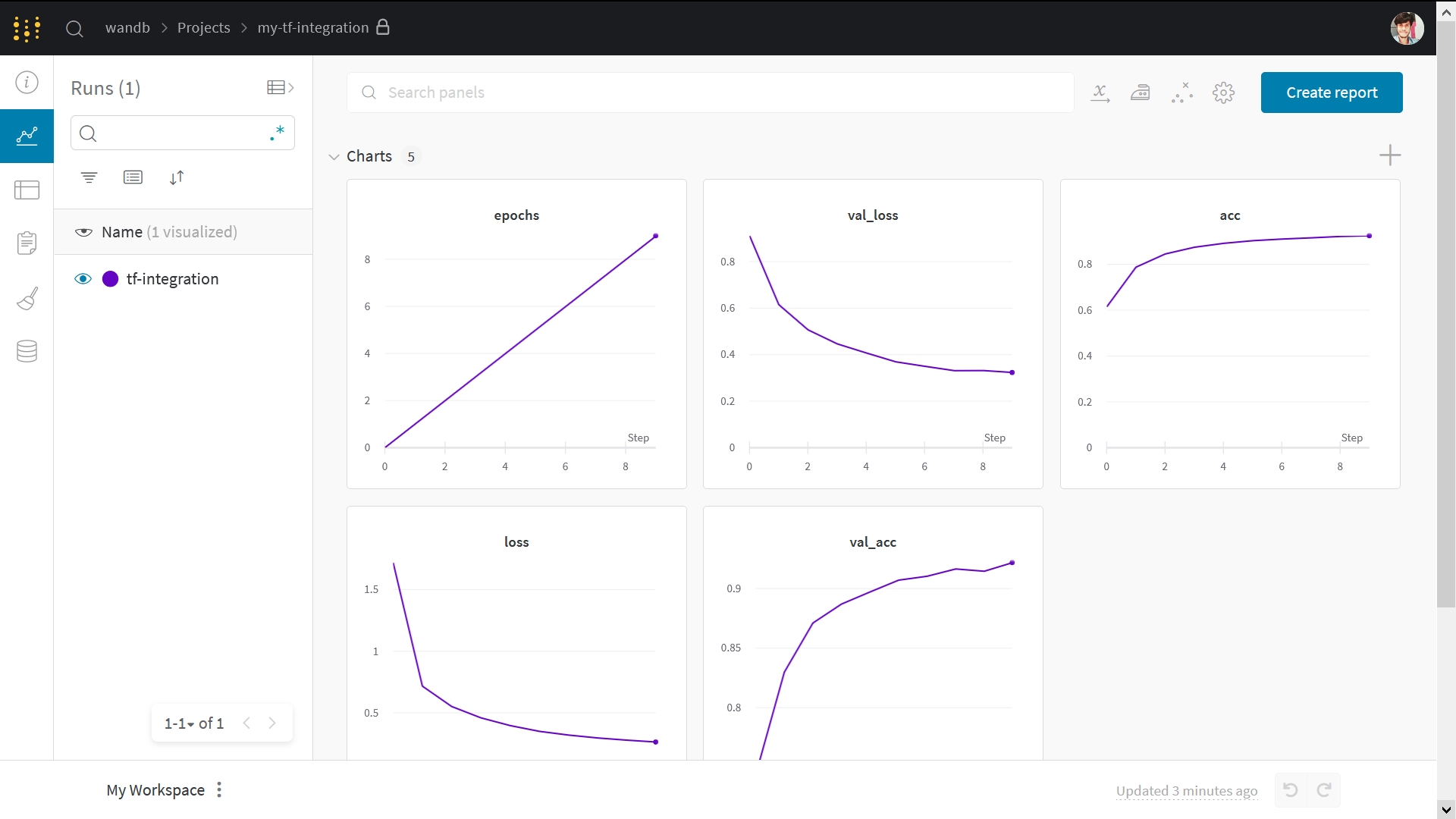
Note: Sections starting with Step are all you need to integrate W&B into existing code. The rest is just a standard MNIST example.
Install, Import, Login
Install W&B
Import W&B and login
Side note: If this is your first time using W&B or you are not logged in, the link that appears after running
wandb.login()
will take you to sign-up/login page. Signing up is as easy as one click.
Prepare Dataset
Define the Model and the Training Loop
Add wandb.log
to your training loop
Run Training
Call wandb.init
to start a run
This lets us know you’re launching an experiment, so we can give it a unique ID and a dashboard.
Check out the official documentation
Visualize Results
Click on the run page link above to see your live results.
Sweep 101
Use Weights & Biases Sweeps to automate hyperparameter optimization and explore the space of possible models.
Check out Hyperparameter Optimization in TensorFlow using W&B Sweeps
Benefits of using W&B Sweeps
- Quick setup: With just a few lines of code you can run W&B sweeps.
- Transparent: We cite all the algorithms we’re using, and our code is open source.
- Powerful: Our sweeps are completely customizable and configurable. You can launch a sweep across dozens of machines, and it’s just as easy as starting a sweep on your laptop.
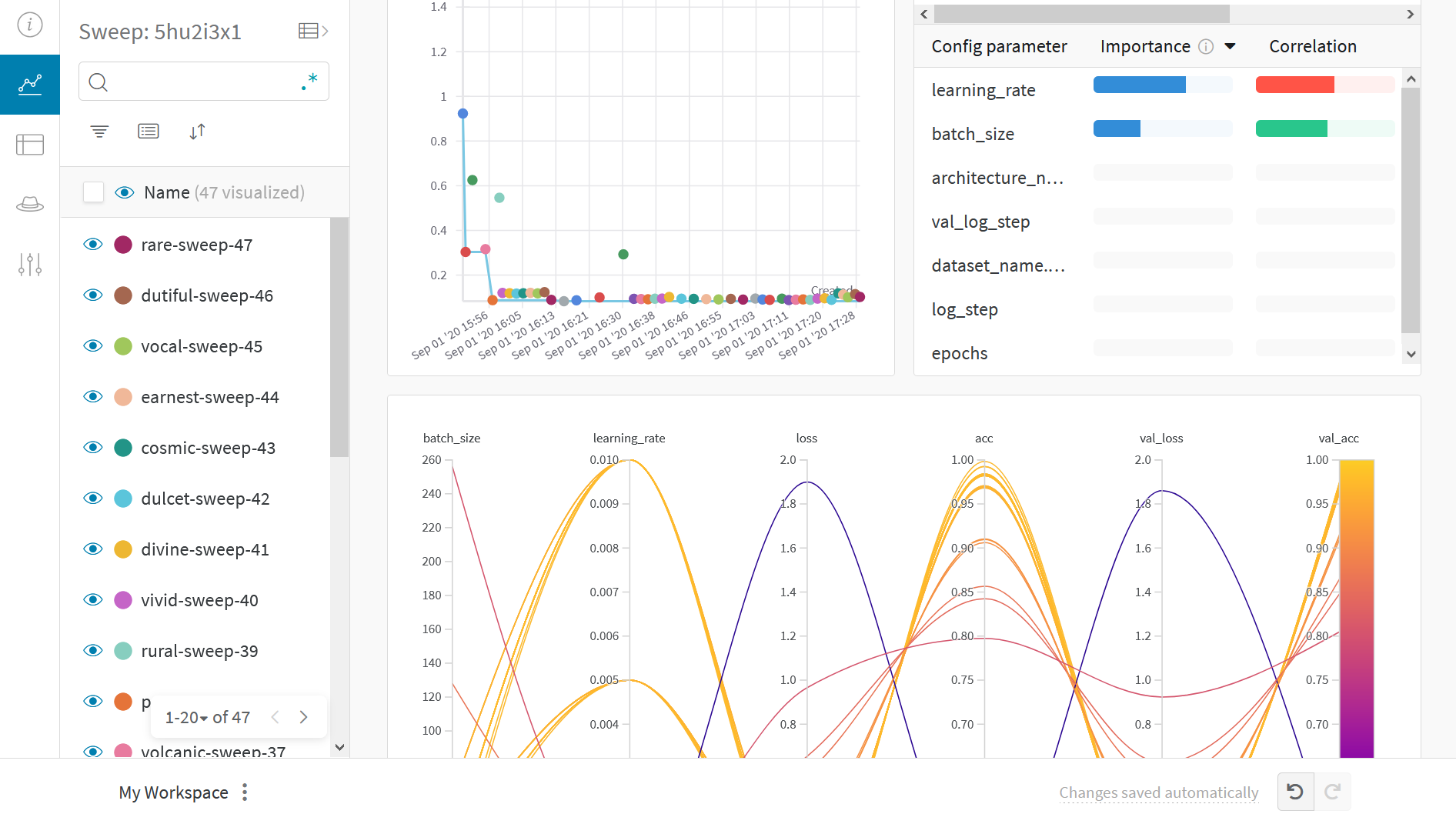
Example Gallery
See examples of projects tracked and visualized with W&B in our gallery of examples, Fully Connected →
Best Practices
- Projects: Log multiple runs to a project to compare them.
wandb.init(project="project-name")
- Groups: For multiple processes or cross validation folds, log each process as a runs and group them together.
wandb.init(group="experiment-1")
- Tags: Add tags to track your current baseline or production model.
- Notes: Type notes in the table to track the changes between runs.
- Reports: Take quick notes on progress to share with colleagues and make dashboards and snapshots of your ML projects.
Advanced Setup
- Environment variables: Set API keys in environment variables so you can run training on a managed cluster.
- Offline mode
- On-prem: Install W&B in a private cloud or air-gapped servers in your own infrastructure. We have local installations for everyone from academics to enterprise teams.
- Artifacts: Track and version models and datasets in a streamlined way that automatically picks up your pipeline steps as you train models.
5 - TensorFlow Sweeps
Use W&B for machine learning experiment tracking, dataset versioning, and project collaboration.
Use W&B Sweeps to automate hyperparameter optimization and explore model possibilities with interactive dashboards:
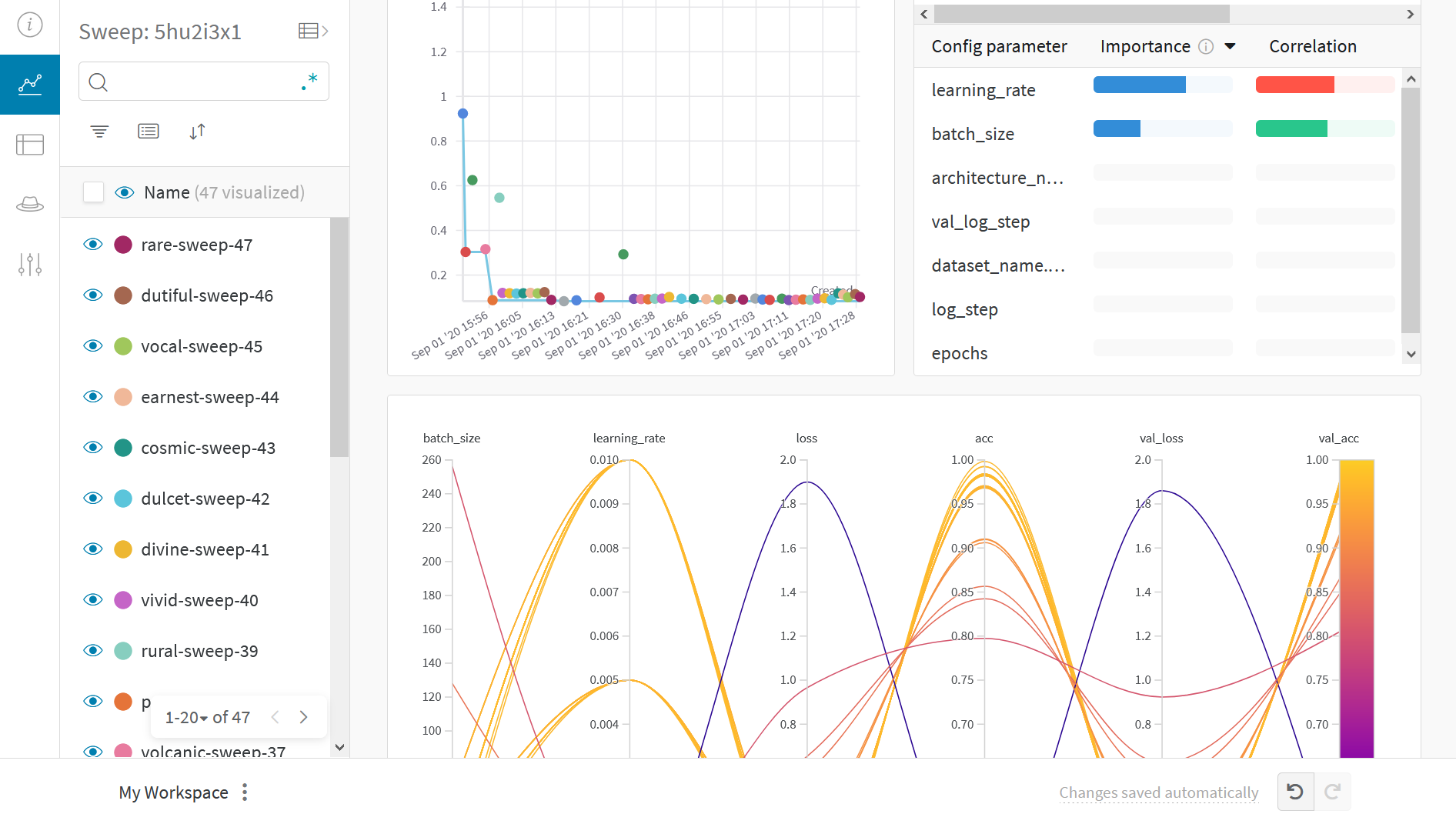
Why use sweeps
- Quick setup: Run W&B sweeps with a few lines of code.
- Transparent: The project cites all algorithms used, and the code is open source.
- Powerful: Sweeps provide customization options and can run on multiple machines or a laptop with ease.
For more information, see the Sweep documentation.
What this notebook covers
- Steps to start with W&B Sweep and a custom training loop in TensorFlow.
- Finding best hyperparameters for image classification tasks.
Note: Sections starting with Step show necessary code to perform a hyperparameter sweep. The rest sets up a simple example.
Install, import, and log in
Install W&B
Import W&B and log in
wandb.login()
directs to the sign-up/login page.Prepare dataset
Build a classifier MLP
Write a training loop
Configure the sweep
Steps to configure the sweep:
- Define the hyperparameters to optimize
- Choose the optimization method:
random
,grid
, orbayes
- Set a goal and metric for
bayes
, like minimizingval_loss
- Use
hyperband
for early termination of performing runs
See more in the W&B Sweeps documentation.
Wrap the training loop
Create a function, like sweep_train
,
which uses wandb.config
to set hyperparameters before calling train
.
Initialize sweep and run personal digital assistant
Limit the number of runs with the count
parameter. Set to 10 for quick execution. Increase as needed.
Visualize results
Click on the Sweep URL link preceding to view live results.
Example gallery
Explore projects tracked and visualized with W&B in the Gallery.
Best practices
- Projects: Log multiple runs to a project to compare them.
wandb.init(project="project-name")
- Groups: Log each process as a run for multiple processes or cross-validation folds, and group them.
wandb.init(group='experiment-1')
- Tags: Use tags to track your baseline or production model.
- Notes: Enter notes in the table to track changes between runs.
- Reports: Use reports for progress notes, sharing with colleagues, and creating ML project dashboards and snapshots.
Advanced setup
- Environment variables: Set API keys for training on a managed cluster.
- Offline mode
- On-prem: Install W&B in a private cloud or air-gapped servers in your infrastructure. Local installations suit academics and enterprise teams.
6 - 3D brain tumor segmentation with MONAI
This tutorial demonstrates how to construct a training workflow of multi-labels 3D brain tumor segmentation task using MONAI and use experiment tracking and data visualization features of Weights & Biases. The tutorial contains the following features:
- Initialize a Weights & Biases run and synchronize all configs associated with the run for reproducibility.
- MONAI transform API:
- MONAI Transforms for dictionary format data.
- How to define a new transform according to MONAI
transforms
API. - How to randomly adjust intensity for data augmentation.
- Data Loading and Visualization:
- Load
Nifti
image with metadata, load a list of images and stack them. - Cache IO and transforms to accelerate training and validation.
- Visualize the data using
wandb.Table
and interactive segmentation overlay on Weights & Biases.
- Load
- Training a 3D
SegResNet
model- Using the
networks
,losses
, andmetrics
APIs from MONAI. - Training the 3D
SegResNet
model using a PyTorch training loop. - Track the training experiment using Weights & Biases.
- Log and version model checkpoints as model artifacts on Weights & Biases.
- Using the
- Visualize and compare the predictions on the validation dataset using
wandb.Table
and interactive segmentation overlay on Weights & Biases.
Setup and Installation
First, install the latest version of both MONAI and Weights and Biases.
Then, authenticate the Colab instance to use W&B.
Initialize a W&B Run
Start a new W&B run to start tracking the experiment.
Use of proper config system is a recommended best practice for reproducible machine learning. You can track the hyperparameters for every experiment using W&B.
You also need to set the random seed for modules to enable or turn off deterministic training.
Data Loading and Transformation
Here, use the monai.transforms
API to create a custom transform that converts the multi-classes labels into multi-labels segmentation task in one-hot format.
Next, set up transforms for training and validation datasets respectively.
The Dataset
The dataset used for this experiment comes from http://medicaldecathlon.com/. It uses multi-modal multi-site MRI data (FLAIR, T1w, T1gd, T2w) to segment Gliomas, necrotic/active tumour, and oedema. The dataset consists of 750 4D volumes (484 Training + 266 Testing).
Use the DecathlonDataset
to automatically download and extract the dataset. It inherits MONAI CacheDataset
which enables you to set cache_num=N
to cache N
items for training and use the default arguments to cache all the items for validation, depending on your memory size.
train_transform
to the train_dataset
, apply val_transform
to both the training and validation datasets. This is because, before training, you would be visualizing samples from both the splits of the dataset.Visualizing the Dataset
Weights & Biases supports images, video, audio, and more. You can log rich media to explore your results and visually compare our runs, models, and datasets. Use the segmentation mask overlay system to visualize our data volumes. To log segmentation masks in tables, you must provide a wandb.Image
object for each row in the table.
An example is provided in the pseudocode below:
Now write a simple utility function that takes a sample image, label, wandb.Table
object and some associated metadata and populate the rows of a table that would be logged to the Weights & Biases dashboard.
Next, define the wandb.Table
object and what columns it consists of so that it can populate with the data visualizations.
Then, loop over the train_dataset
and val_dataset
respectively to generate the visualizations for the data samples and populate the rows of the table which to log to the dashboard.
The data appears on the W&B dashboard in an interactive tabular format. We can see each channel of a particular slice from a data volume overlaid with the respective segmentation mask in each row. You can write Weave queries to filter the data on the table and focus on one particular row.
![]() |
---|
An example of logged table data. |
Open an image and see how you can interact with each of the segmentation masks using the interactive overlay.
![]() |
---|
*An example of visualized segmentation maps. |
Loading the Data
Create the PyTorch DataLoaders for loading the data from the datasets. Before creating the DataLoaders, set the transform
for train_dataset
to train_transform
to pre-process and transform the data for training.
Creating the Model, Loss, and Optimizer
This tutorial crates a SegResNet
model based on the paper 3D MRI brain tumor segmentation using auto-encoder regularization. The SegResNet
model that comes implemented as a PyTorch Module as part of the monai.networks
API as well as an optimizer and learning rate scheduler.
Define the loss as multi-label DiceLoss
using the monai.losses
API and the corresponding dice metrics using the monai.metrics
API.
Define a small utility for mixed-precision inference. This will be useful during the validation step of the training process and when you want to run the model after training.
Training and Validation
Before training, define the metric properties which will later be logged with wandb.log()
for tracking the training and validation experiments.
Execute Standard PyTorch Training Loop
Instrumenting the code with wandb.log
not only enables tracking all metrics associated with the training and validation process, but also the all system metrics (our CPU and GPU in this case) on the W&B dashboard.
![]() |
---|
An example of training and validation process tracking on W&B. |
Navigate to the artifacts tab in the W&B run dashboard to access the different versions of model checkpoint artifacts logged during training.
![]() |
---|
An example of model checkpoints logging and versioning on W&B. |
Inference
Using the artifacts interface, you can select which version of the artifact is the best model checkpoint, in this case, the mean epoch-wise training loss. You can also explore the entire lineage of the artifact and use the version that you need.
![]() |
---|
An example of model artifact tracking on W&B. |
Fetch the version of the model artifact with the best epoch-wise mean training loss and load the checkpoint state dictionary to the model.
Visualizing Predictions and Comparing with the Ground Truth Labels
Create another utility function to visualize the predictions of the pre-trained model and compare them with the corresponding ground-truth segmentation mask using the interactive segmentation mask overlay,.
Log the prediction results to the prediction table.
Use the interactive segmentation mask overlay to analyze and compare the predicted segmentation masks and the ground-truth labels for each class.
![]() |
---|
An example of predictions and ground-truth visualization on W&B. |
Acknowledgements and more resources
7 - Keras
Use Weights & Biases for machine learning experiment tracking, dataset versioning, and project collaboration.
This Colab notebook introduces the WandbMetricsLogger
callback. Use this callback for Experiment Tracking. It will log your training and validation metrics along with system metrics to Weights and Biases.
Setup and Installation
First, let us install the latest version of Weights and Biases. We will then authenticate this colab instance to use W&B.
If this is your first time using W&B or you are not logged in, the link that appears after running wandb.login()
will take you to sign-up/login page. Signing up for a free account is as easy as a few clicks.
Hyperparameters
Use of proper config system is a recommended best practice for reproducible machine learning. We can track the hyperparameters for every experiment using W&B. In this colab we will be using simple Python dict
as our config system.
Dataset
In this colab, we will be using CIFAR100 dataset from TensorFlow Dataset catalog. We aim to build a simple image classification pipeline using TensorFlow/Keras.
Model
Compile Model
Train
8 - Keras models
Use Weights & Biases for machine learning experiment tracking, dataset versioning, and project collaboration.
This Colab notebook introduces the WandbModelCheckpoint
callback. Use this callback to log your model checkpoints to Weight and Biases Artifacts.
Setup and Installation
First, let us install the latest version of Weights and Biases. We will then authenticate this colab instance to use W&B.
If this is your first time using W&B or you are not logged in, the link that appears after running wandb.login()
will take you to sign-up/login page. Signing up for a free account is as easy as a few clicks.
Hyperparameters
Use of proper config system is a recommended best practice for reproducible machine learning. We can track the hyperparameters for every experiment using W&B. In this colab we will be using simple Python dict
as our config system.
Dataset
In this colab, we will be using CIFAR100 dataset from TensorFlow Dataset catalog. We aim to build a simple image classification pipeline using TensorFlow/Keras.
Model
Compile Model
Train
9 - Keras tables
Use Weights & Biases for machine learning experiment tracking, dataset versioning, and project collaboration.
This Colab notebook introduces the WandbEvalCallback
which is an abstract callback that be inherited to build useful callbacks for model prediction visualization and dataset visualization.
Setup and Installation
First, let us install the latest version of Weights and Biases. We will then authenticate this colab instance to use W&B.
If this is your first time using W&B or you are not logged in, the link that appears after running wandb.login()
will take you to sign-up/login page. Signing up for a free account is as easy as a few clicks.
Hyperparameters
Use of proper config system is a recommended best practice for reproducible machine learning. We can track the hyperparameters for every experiment using W&B. In this colab we will be using simple Python dict
as our config system.
Dataset
In this colab, we will be using CIFAR100 dataset from TensorFlow Dataset catalog. We aim to build a simple image classification pipeline using TensorFlow/Keras.
AUTOTUNE = tf.data.AUTOTUNE
def parse_data(example):
# Get image
image = example["image"]
# image = tf.image.convert_image_dtype(image, dtype=tf.float32)
# Get label
label = example["label"]
label = tf.one_hot(label, depth=configs["num_classes"])
return image, label
def get_dataloader(ds, configs, dataloader_type="train"):
dataloader = ds.map(parse_data, num_parallel_calls=AUTOTUNE)
if dataloader_type=="train":
dataloader = dataloader.shuffle(configs["shuffle_buffer"])
dataloader = (
dataloader
.batch(configs["batch_size"])
.prefetch(AUTOTUNE)
)
return dataloader
Model
Compile Model
WandbEvalCallback
The WandbEvalCallback
is an abstract base class to build Keras callbacks for primarily model prediction visualization and secondarily dataset visualization.
This is a dataset and task agnostic abstract callback. To use this, inherit from this base callback class and implement the add_ground_truth
and add_model_prediction
methods.
The WandbEvalCallback
is a utility class that provides helpful methods to:
- create data and prediction
wandb.Table
instances, - log data and prediction Tables as
wandb.Artifact
, - logs the data table
on_train_begin
, - logs the prediction table
on_epoch_end
.
As an example, we have implemented WandbClfEvalCallback
below for an image classification task. This example callback:
- logs the validation data (
data_table
) to W&B, - performs inference and logs the prediction (
pred_table
) to W&B on every epoch end.
How the memory footprint is reduced
We log the data_table
to W&B when the on_train_begin
method is ivoked. Once it’s uploaded as a W&B Artifact, we get a reference to this table which can be accessed using data_table_ref
class variable. The data_table_ref
is a 2D list that can be indexed like self.data_table_ref[idx][n]
where idx
is the row number while n
is the column number. Let’s see the usage in the example below.
Train
10 - XGBoost Sweeps
Use Weights & Biases for machine learning experiment tracking, dataset versioning, and project collaboration.
Squeezing the best performance out of tree-based models requires
selecting the right hyperparameters.
How many early_stopping_rounds
? What should the max_depth
of a tree be?
Searching through high dimensional hyperparameter spaces to find the most performant model can get unwieldy very fast. Hyperparameter sweeps provide an organized and efficient way to conduct a battle royale of models and crown a winner. They enable this by automatically searching through combinations of hyperparameter values to find the most optimal values.
In this tutorial we’ll see how you can run sophisticated hyperparameter sweeps on XGBoost models in 3 easy steps using Weights and Biases.
For a teaser, check out the plots below:
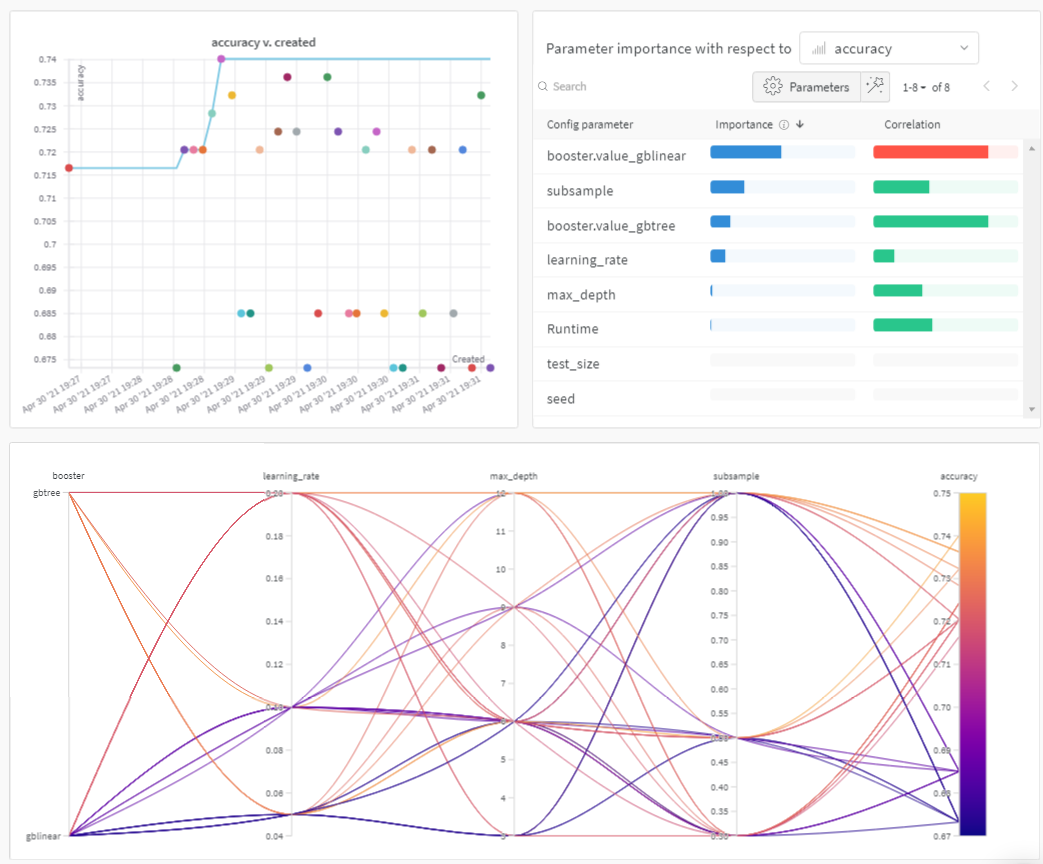
Sweeps: An Overview
Running a hyperparameter sweep with Weights & Biases is very easy. There are just 3 simple steps:
-
Define the sweep: we do this by creating a dictionary-like object that specifies the sweep: which parameters to search through, which search strategy to use, which metric to optimize.
-
Initialize the sweep: with one line of code we initialize the sweep and pass in the dictionary of sweep configurations:
sweep_id = wandb.sweep(sweep_config)
-
Run the sweep agent: also accomplished with one line of code, we call w
andb.agent()
and pass thesweep_id
along with a function that defines your model architecture and trains it:wandb.agent(sweep_id, function=train)
That’s all there is to running a hyperparameter sweep.
In the notebook below, we’ll walk through these 3 steps in more detail.
We highly encourage you to fork this notebook, tweak the parameters, or try the model with your own dataset.
Resources
1. Define the Sweep
Weights & Biases sweeps give you powerful levers to configure your sweeps exactly how you want them, with just a few lines of code. The sweeps config can be defined as a dictionary or a YAML file.
Let’s walk through some of them together:
- Metric: This is the metric the sweeps are attempting to optimize. Metrics can take a
name
(this metric should be logged by your training script) and agoal
(maximize
orminimize
). - Search Strategy: Specified using the
"method"
key. We support several different search strategies with sweeps. - Grid Search: Iterates over every combination of hyperparameter values.
- Random Search: Iterates over randomly chosen combinations of hyperparameter values.
- Bayesian Search: Creates a probabilistic model that maps hyperparameters to probability of a metric score, and chooses parameters with high probability of improving the metric. The objective of Bayesian optimization is to spend more time in picking the hyperparameter values, but in doing so trying out fewer hyperparameter values.
- Parameters: A dictionary containing the hyperparameter names, and discrete values, a range, or distributions from which to pull their values on each iteration.
For details, see the list of all sweep configuration options.
2. Initialize the Sweep
Calling wandb.sweep
starts a Sweep Controller –
a centralized process that provides settings of the parameters
to any who query it
and expects them to return performance on metrics
via wandb
logging.
Define your training process
Before we can run the sweep, we need to define a function that creates and trains the model – the function that takes in hyperparameter values and spits out metrics.
We’ll also need wandb
to be integrated into our script.
There’s three main components:
wandb.init()
: Initialize a new W&B run. Each run is single execution of the training script.wandb.config
: Save all your hyperparameters in a config object. This lets you use our app to sort and compare your runs by hyperparameter values.wandb.log()
: Logs metrics and custom objects, such as images, videos, audio files, HTML, plots, or point clouds.
We also need to download the data:
3. Run the Sweep with an agent
Now, we call wandb.agent
to start up our sweep.
You can call wandb.agent
on any machine where you’re logged into W&B that has
- the
sweep_id
, - the dataset and
train
function
and that machine will join the sweep.
Note: a
random
sweep will by defauly run forever, trying new parameter combinations until the cows come home – or until you turn the sweep off from the app UI. You can prevent this by providing the totalcount
of runs you’d like theagent
to complete.
Visualize your results
Now that your sweep is finished, it’s time to look at the results.
Weights & Biases will generate a number of useful plots for you automatically.
Parallel coordinates plot
This plot maps hyperparameter values to model metrics. It’s useful for honing in on combinations of hyperparameters that led to the best model performance.
This plot seems to indicate that using a tree as our learner slightly, but not mind-blowingly, outperforms using a simple linear model as our learner.
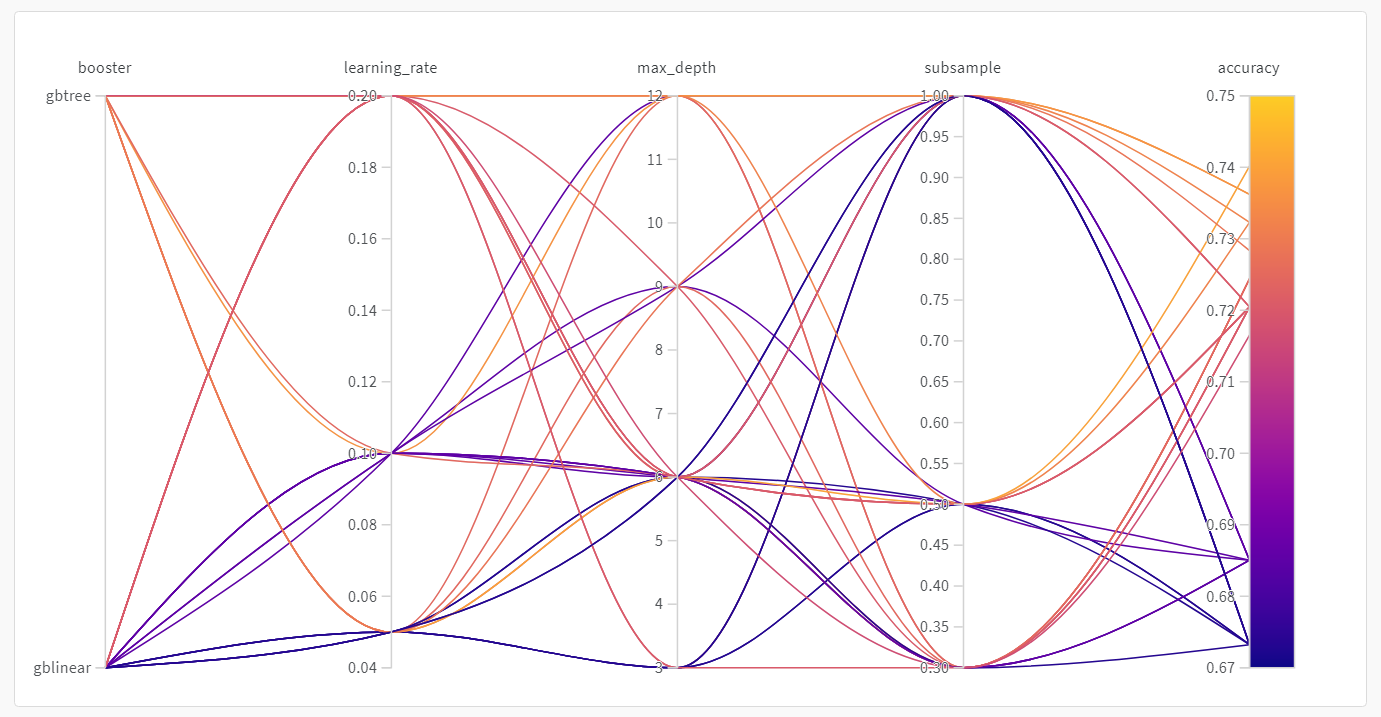
Hyperparameter importance plot
The hyperparameter importance plot shows which hyperparameter values had the biggest impact on your metrics.
We report both the correlation (treating it as a linear predictor) and the feature importance (after training a random forest on your results) so you can see which parameters had the biggest effect and whether that effect was positive or negative.
Reading this chart, we see quantitative confirmation
of the trend we noticed in the parallel coordinates chart above:
the largest impact on validation accuracy came from the choice of
learner, and the gblinear
learners were generally worse than gbtree
learners.
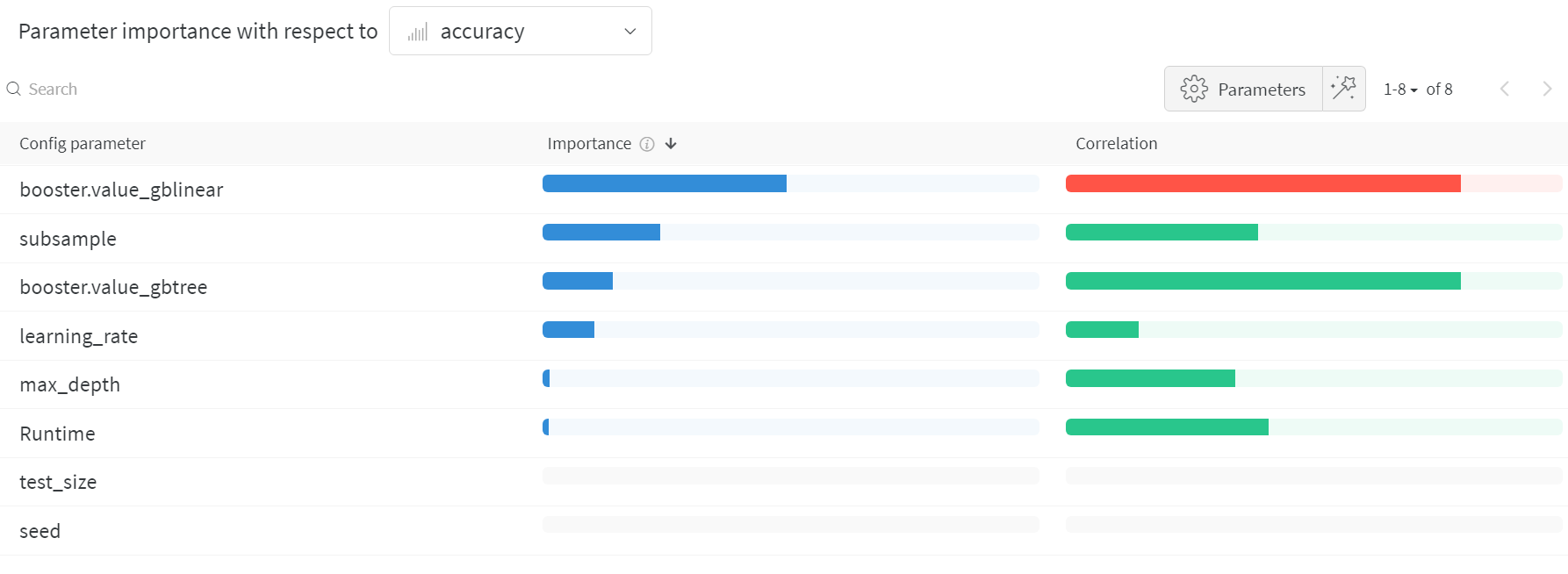
These visualizations can help you save both time and resources running expensive hyperparameter optimizations by honing in on the parameters (and value ranges) that are the most important, and thereby worthy of further exploration.